Beginning C# 6 Programming with Visual Studio 2015
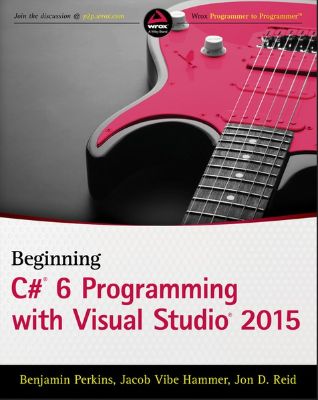
Lýsing:
Get started with Visual C# programming with this great beginner's guide Beginning C# 6 Programming with Visual Studio 2015 provides step-by-step directions for programming with C# in the. NET framework. Beginning with programming essentials, such as variables, flow control, and object-oriented programming, this authoritative text moves into more complicated topics, such as web and Windows programming and data access within both database and XML environments.
After your introduction to each of the chapters, you are invited to apply your newfound knowledge in Try it Out sections, which reinforce learning and help you understand the practical applications of the new concepts you have explored. Through this approach, you can write useful programming code following each of the steps that you explore in this essential text. Discover the basics of programming with C#, such as variables, expressions, flow control, and functions Discuss how to keep your program running smoothly through debugging and error handling Understand how to navigate your way through key programming elements, such as classes, class members, collections, comparisons, and conversions Explore object-oriented programming, web programming, and Windows programming Beginning C# 6 Programming with Visual Studio 2015 is a fundamental resource for any programmers who are new to the C# language.
Annað
- Höfundar: Benjamin Perkins, Jacob Vibe Hammer, Jon D. Reid
- Útgáfa:1
- Útgáfudagur: 2015-12-21
- Blaðsíður: 840
- Hægt að prenta út 10 bls.
- Hægt að afrita 2 bls.
- Format:Page Fidelity
- ISBN 13: 9781119096566
- Print ISBN: 9781119096689
- ISBN 10: 1119096561
Efnisyfirlit
- Title Page
- Copyright
- Contents
- Introduction
- Part I: The OOP Language
- Chapter 1: Introducing C#
- What Is the .NET Framework?
- What’s in the .NET Framework?
- Writing Applications Using the .NET Framework
- What Is C#?
- Applications You Can Write with C#
- C# in this Book
- Visual Studio 2015
- Visual Studio Express 2015 Products
- Solutions
- What Is the .NET Framework?
- Chapter 1: Introducing C#
- Chapter 2: Writing a C# Program
- The Visual Studio 2015 Development Environment
- Console Applications
- The Solution Explorer
- The Properties Window
- The Error List Window
- Desktop Applications
- Chapter 3: Variables and Expressions
- Basic C# Syntax
- Basic C# Console Application Structure
- Variables
- Simple Types
- Variable Naming
- Literal Values
- Expressions
- Mathematical Operators
- Assignment Operators
- Operator Precedence
- Namespaces
- Boolean Logic
- Boolean Bitwise and Assignment Operators
- Operator Precedence Updated
- Branching
- The Ternary Operator
- The if Statement
- The switch Statement
- Looping
- do Loops
- while Loops
- for Loops
- Interrupting Loops
- Infinite Loops
- Type Conversion
- Implicit Conversions
- Explicit Conversions
- Explicit Conversions Using the Convert Commands
- Complex Variable Types
- Enumerations
- Structs
- Arrays
- String Manipulation
- Defining and Using Functions
- Return Values
- Parameters
- Variable Scope
- Variable Scope in Other Structures
- Parameters and Return Values versus Global Data
- The Main() Function
- Struct Functions
- Overloading Functions
- Using Delegates
- Debugging in Visual Studio
- Debugging in Nonbreak (Normal) Mode
- Debugging in Break Mode
- Error Handling
- try…catch…finally
- Listing and Configuring Exceptions
- What Is Object-Oriented Programming?
- What Is an Object?
- Everything’s an Object
- The Life Cycle of an Object
- Static and Instance Class Members
- OOP Techniques
- Interfaces
- Inheritance
- Polymorphism
- Relationships between Objects
- Operator Overloading
- Events
- Reference Types versus Value Types
- OOP in Desktop Applications
- Class Definitions in C#
- Interface Definitions
- System.Object
- Constructors and Destructors
- Constructor Execution Sequence
- OOP Tools in Visual Studio
- The Class View Window
- The Object Browser
- Adding Classes
- Class Diagrams
- Class Library Projects
- Interfaces versus Abstract Classes
- Struct Types
- Shallow Copying versus Deep Copying
- Member Definitions
- Defining Fields
- Defining Methods
- Defining Properties
- Refactoring Members
- Automatic Properties
- Additional Class Member Topics
- Hiding Base Class Methods
- Calling Overridden or Hidden Base Class Methods
- Using Nested Type Definitions
- Interface Implementation
- Implementing Interfaces in Classes
- Partial Class Definitions
- Partial Method Definitions
- Example Application
- Planning the Application
- Writing the Class Library
- A Client Application for the Class Library
- The Call Hierarchy Window
- Collections
- Using Collections
- Defining Collections
- Indexers
- Adding a Cards Collection to CardLib
- Keyed Collections and IDictionary
- Iterators
- Iterators and Collections
- Deep Copying
- Adding Deep Copying to CardLib
- Comparisons
- Type Comparisons
- Value Comparisons
- Conversions
- Overloading Conversion Operators
- The as Operator
- What Are Generics?
- Using Generics
- Nullable Types
- The System.Collections.Generic Namespace
- Defining Generic Types
- Defining Generic Classes
- Defining Generic Interfaces
- Defining Generic Methods
- Defining Generic Delegates
- Variance
- Covariance
- Contravariance
- The : : Operator and the Global Namespace Qualifier
- Custom Exceptions
- Adding Custom Exceptions to CardLib
- Events
- What Is an Event?
- Handling Events
- Defining Events
- Expanding and Using CardLib
- Attributes
- Reading Attributes
- Creating Attributes
- Initializers
- Object Initializers
- Collection Initializers
- Type Inference
- Anonymous Types
- Dynamic Lookup
- The dynamic Type
- Advanced Method Parameters
- Optional Parameters
- Named Parameters
- Lambda Expressions
- Anonymous Methods Recap
- Lambda Expressions for Anonymous Methods
- Lambda Expression Parameters
- Lambda Expression Statement Bodies
- Lambda Expressions as Delegates and Expression Trees
- Lambda Expressions and Collections
- Chapter 14: Basic Desktop Programming
- XAML
- Separation of Concerns
- XAML in Action
- The Playground
- WPF Controls
- Properties
- Events
- Control Layout
- Stack Order
- Alignment, Margins, Padding, and Dimensions
- Border
- Canvas
- DockPanel
- StackPanel
- WrapPanel
- Grid
- The Game Client
- The About Window
- The Options Window
- Data Binding
- Starting a Game with the ListBox Control
- XAML
- The Main Window
- The Menu Control
- Routed Commands with Menus
- Creating and Styling Controls
- Styles
- Templates
- Value Converters
- Triggers
- Animations
- WPF User Controls
- Implementing Dependency Properties
- Putting It All Together
- Refactoring the Domain Model
- The View Models
- Completing the Game
- Chapter 16: Basic Cloud Programming
- The Cloud, Cloud Computing, and the Cloud Optimized Stack
- Cloud Patterns and Best Practices
- Using Microsoft Azure C# Libraries to Create a Storage Container
- Creating an ASP.NET 4.6 Web Site That Uses the Storage Container
- Chapter 17: Advanced Cloud Programing and Deployment
- Creating an ASP.NET Web API
- Deploying and Consuming an ASP.NET Web API on Microsoft Azure
- Scaling an ASP.NET Web API on Microsoft Azure
- Chapter 18: Files
- File Classes for Input and Output
- The File and Directory Classes
- The FileInfo Class
- The DirectoryInfo Class
- Path Names and Relative Paths
- Streams
- Classes for Using Streams
- The FileStream Object
- The StreamWriter Object
- The StreamReader Object
- Asynchronous File Access
- Reading and Writing Compressed Files
- Monitoring the File System
- File Classes for Input and Output
- Chapter 19: XML and JSON
- XML Basics
- JSON Basics
- XML Schemas
- XML Document Object Model
- The XmlDocument Class
- The XmlElement Class
- Changing the Values of Nodes
- Converting XML to JSON
- Searching XML with XPath
- Chapter 20: LINQ
- LINQ to XML
- LINQ to XML Functional Constructors
- Working with XML Fragments
- LINQ Providers
- LINQ Query Syntax
- Declaring a Variable for Results Using the var Keyword
- Specifying the Data Source: from Clause
- Specify Condition: where Clause
- Selecting Items: select Clause
- Finishing Up: Using the foreach Loop
- Deferred Query Execution
- LINQ Method Syntax
- LINQ Extension Methods
- Query Syntax versus Method Syntax
- Lambda Expressions
- Ordering Query Results
- Understanding the orderby Clause
- Querying a Large Data Set
- Using Aggregate Operators
- Using the Select Distinct Query
- Ordering by Multiple Levels
- Using Group Queries
- Using Joins
- LINQ to XML
- Chapter 21: Databases
- Using Databases
- Installing SQL Server Express
- Entity Framework
- A Code First Database
- But Where Is My Database?
- Navigating Database Relationships
- Handling Migrations
- Creating and Querying XML from an Existing Database
- Chapter 22: Windows Communication Foundation
- What Is WCF?
- WCF Concepts
- WCF Communication Protocols
- Addresses, Endpoints, and Bindings
- Contracts
- Message Patterns
- Behaviors
- Hosting
- WCF Programming
- The WCF Test Client
- Defining WCF Service Contracts
- Self-Hosted WCF Services
- Getting Started
- Universal Apps
- App Concepts and Design
- Screen Orientation
- Menus and Toolbars
- Tiles and Badges
- App Lifetime
- Lock Screen Apps
- App Development
- Adaptive Displays
- Sandboxed Apps
- Navigation between Pages
- The CommandBar Control
- Managing State
- Common Elements of Windows Store Apps
- The Windows Store
- Packaging an App
- Creating the Package
UM RAFBÆKUR Á HEIMKAUP.IS
Bókahillan þín er þitt svæði og þar eru bækurnar þínar geymdar. Þú kemst í bókahilluna þína hvar og hvenær sem er í tölvu eða snjalltæki. Einfalt og þægilegt!Rafbók til eignar
Rafbók til eignar þarf að hlaða niður á þau tæki sem þú vilt nota innan eins árs frá því bókin er keypt.
Þú kemst í bækurnar hvar sem er
Þú getur nálgast allar raf(skóla)bækurnar þínar á einu augabragði, hvar og hvenær sem er í bókahillunni þinni. Engin taska, enginn kyndill og ekkert vesen (hvað þá yfirvigt).
Auðvelt að fletta og leita
Þú getur flakkað milli síðna og kafla eins og þér hentar best og farið beint í ákveðna kafla úr efnisyfirlitinu. Í leitinni finnur þú orð, kafla eða síður í einum smelli.
Glósur og yfirstrikanir
Þú getur auðkennt textabrot með mismunandi litum og skrifað glósur að vild í rafbókina. Þú getur jafnvel séð glósur og yfirstrikanir hjá bekkjarsystkinum og kennara ef þeir leyfa það. Allt á einum stað.
Hvað viltu sjá? / Þú ræður hvernig síðan lítur út
Þú lagar síðuna að þínum þörfum. Stækkaðu eða minnkaðu myndir og texta með multi-level zoom til að sjá síðuna eins og þér hentar best í þínu námi.
Fleiri góðir kostir
- Þú getur prentað síður úr bókinni (innan þeirra marka sem útgefandinn setur)
- Möguleiki á tengingu við annað stafrænt og gagnvirkt efni, svo sem myndbönd eða spurningar úr efninu
- Auðvelt að afrita og líma efni/texta fyrir t.d. heimaverkefni eða ritgerðir
- Styður tækni sem hjálpar nemendum með sjón- eða heyrnarskerðingu
- Gerð : 208
- Höfundur : 11936
- Útgáfuár : 2015
- Leyfi : 379